What is a blockchain?
- Bitcoin as First Blockchain Protocol
You might have heard of bitcoin when it comes to Blockchain. Bitcoin was one of the first protocols to use this revolutionary technology called a blockchain.
- Bitcoin white Paper
The Bitcoin white paper was created by the pseudo-anonymous Satoshi Nakamoto and outlined how Bitcoin can make peer-to-peer transactions in a decentralized network. This network was powered by cryptography, decentralism, and allowed people to engage in censorship resistant finance, in a decentralized manner due to its features, which we’ll talk about in a little bit.
- Bitcoin as a Superior Digital Store
People took to this as a superior digital store of value, a better store of value over something like gold, for example, and that’s why you’ll also hear people commonly refer to it as a digital gold similar to gold. There’s a scarce amount or a set amount of bitcoin available on the planet, only so much that you can buy and sell. You can read more about the original vision in the white Paper below;
Blockchain As an Emerging technology and Introduction to Ethereum
Some people, though, saw this technology and wanted to take it a little bit farther, and do even more with this blockchain technology. And a few years later, a man named Vitalik Buterin, released a white paper for a new protocol named Ethereum, which uses this same blockchain infrastructure with an additional feature. And in 2015, he and a number of other co-founders released the project Ethereum, where people could not only make decentralized transactions, but decentralized agreements, decentralized organizations, and all these other ways to interact with each other without a centralized intermediary or centralized governing force. Basically, their idea was to take this thing that made Bitcoin so great, and add decentralized agreements to it, or smart contracts. And in fact, technically, these smart contracts weren’t even really a new idea.
Taking all these basic ideas of how blockchain and Ethereum were introduced to the world and how the smart contracts, decentralized agreements were said to be one of the most revolutionary technologies, we will be looking into one of the most popular Programming languages which is used to create and build smart contracts.
Welcome to Remix (an Online tool for writing solidity code)
By clicking on the link above, you will be redirected to a new web page that is the IDE for writing solidity code. environment. This is where we’re going to learn how to code and interact with our smart contracts. If you want, you can go ahead and accept help out remix. If you’ve never been here before, it’ll give you a quick walkthrough of some of the tools that remix actually has, we’re going to skip over them for now. Remix is such a powerful tool because it has a lot of features that allow us to really see and interact with our smart contracts.
To get a good understanding of the IDE. We will see several things when we first land on the web page.
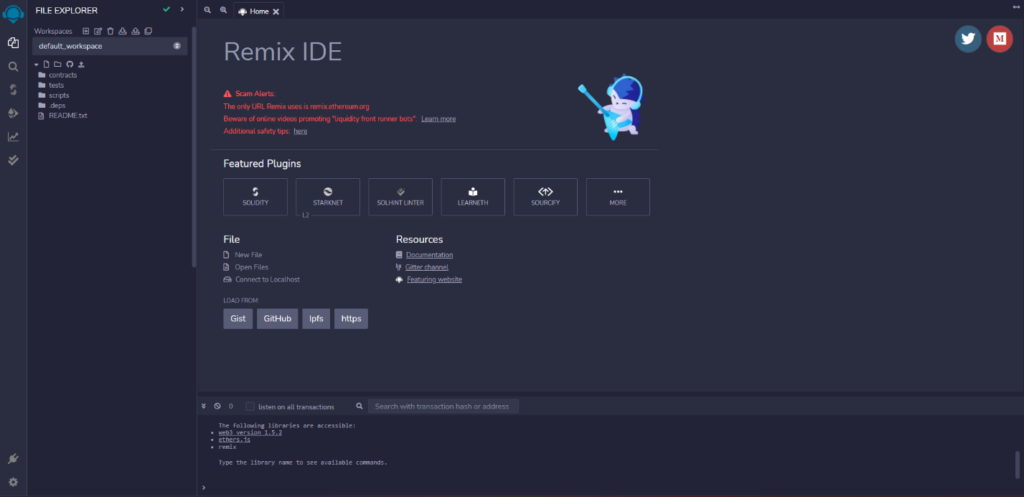
We will be able to notice a development environment like Visual Studio code and other IDEs if you have been working on different projects. We won’t be covering the file structure and other details of the IDE, because they will certainly be clarified once we start writing our first smart contract.
Creating your first Solidity project
Under the Feature Plugins section, you will notice different plugins that are used for different programming languages.
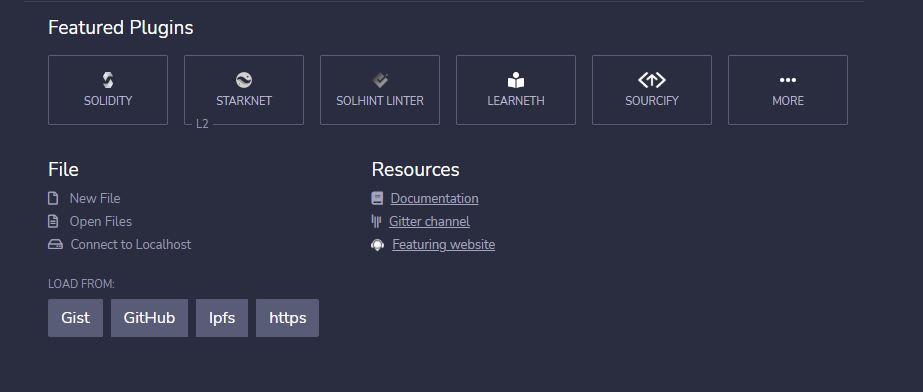
Since we will be working mainly on solidity, we will choose the solidity plugin that is available there. Even if you don’t click the solidity plugin, you will still be able to code solidity smart contracts.
The left-hand side is where we’re going to start to actually interact with our smart contract. The button on the top most of the left is our files or explore directories, remix comes boilerplate with some different contracts, some different scripts, some different tests, and different dependencies.
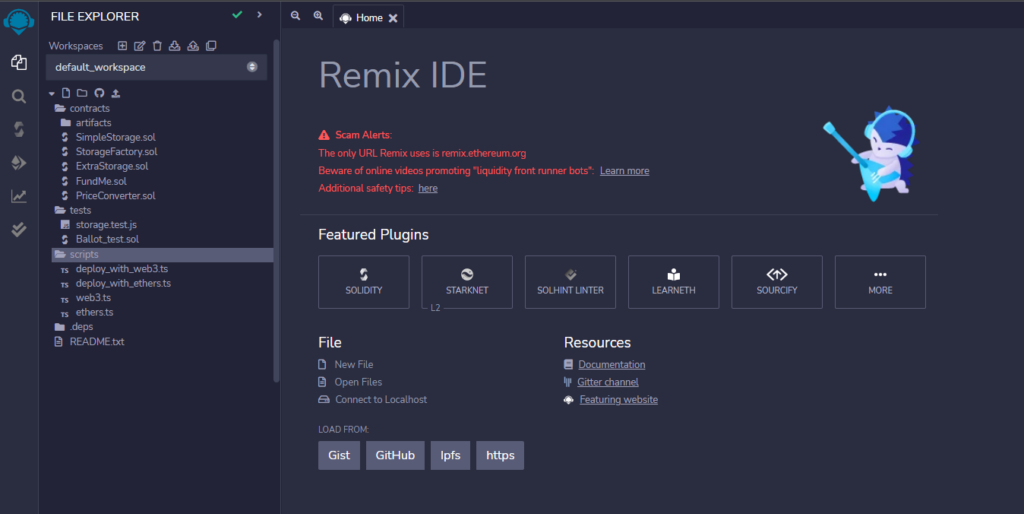
We will be deleting some of the files except the contracts folder and also deleting all the smart contracts that are already provided by Remix. You can always read the READM.TXT for how to write and work with solidity and Remix.
Creating Your first Solidity Smart contract
Click on the smart contract folder and create a new file with (.sol) extension to tell the compiler that it is going to be a solidity file and we are going to code in solidity. You can name your file whatever you would like.
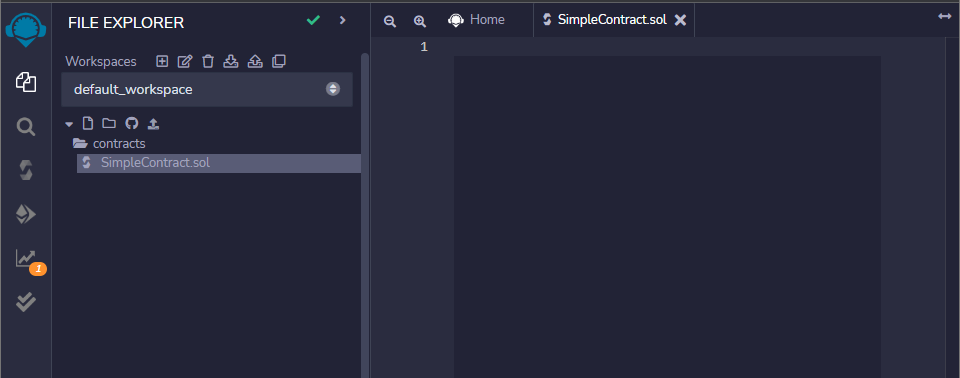
Writing your first line of code in solidity
So, the first thing that you’re going to need in any solidity smart contract is going to be the version of solidity that you’re going to use. And this should always be at the top of your solidity code. You can copy and paste the code below.
pragma solidity 0.8.7;
0.8.7 is the most stable version of the solidity. However, if you are willing to use different versions. Make sure to check the solidity Documentation here;
https://docs.soliditylang.org/en/latest/index.html
You can also write it like below, to let the compiler know what versions will be suitable for the smart contract.
pragma solidity >=0.8.7 <=0.9.0;
Also, at the top of your code, you’re always going to want to put what’s called an SPDX license identifier. This is optional, but some compilers will flag your warning that you don’t have one. This is to make licensing and sharing code a lot easier. We have a link to more about how licenses work here;
//SPDX-License-Identifier:MIT
pragma solidity >=0.8.7 <=0.9.0;
Once you have this much written, we can actually go ahead and write to our compiler tab and scroll down and hit Compile, that little turn thing will go.
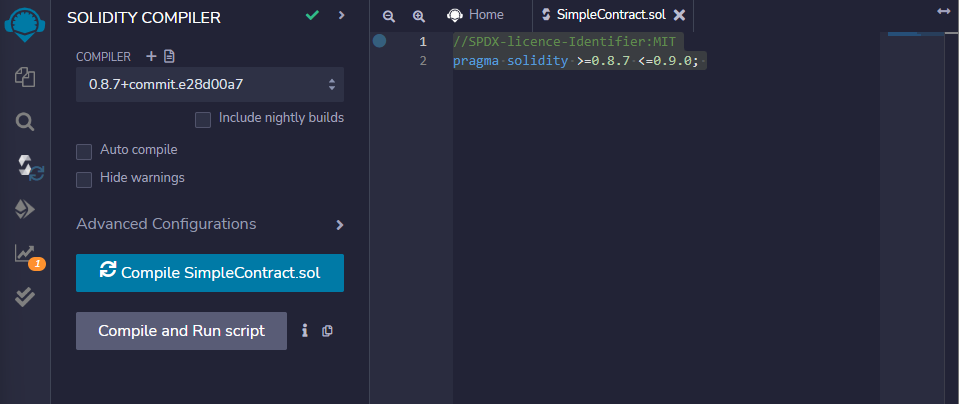
Once we click on the Blue Button with your smart contract name on it. It will compile your smart contract.
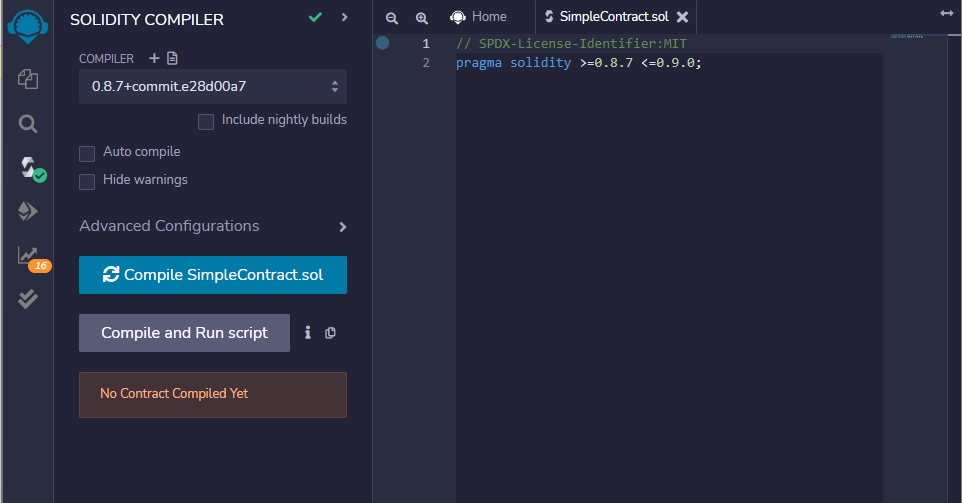
Since we have not written any solidity code for our smart contract, the Compiler says, “No Contract Compiled yet”.
You can also use CMD + S for Mac and CTRL + S for windows to automatically save your file and compile it.
To start defining our contract, we are going to write the following code starting with the word CONTRACT to tell the compiler that the next section is going to define the contract.
//SPDX-License-Identifier:MIT
pragma solidity >=0.8.7 <=0.9.0;
contract SimpleContract {
}
The contract looks similar to “Class” in any object-oriented programming.
Now if you hit save, you will see a green check mark on the compiler button, which means that the code is compiled successfully without any errors.
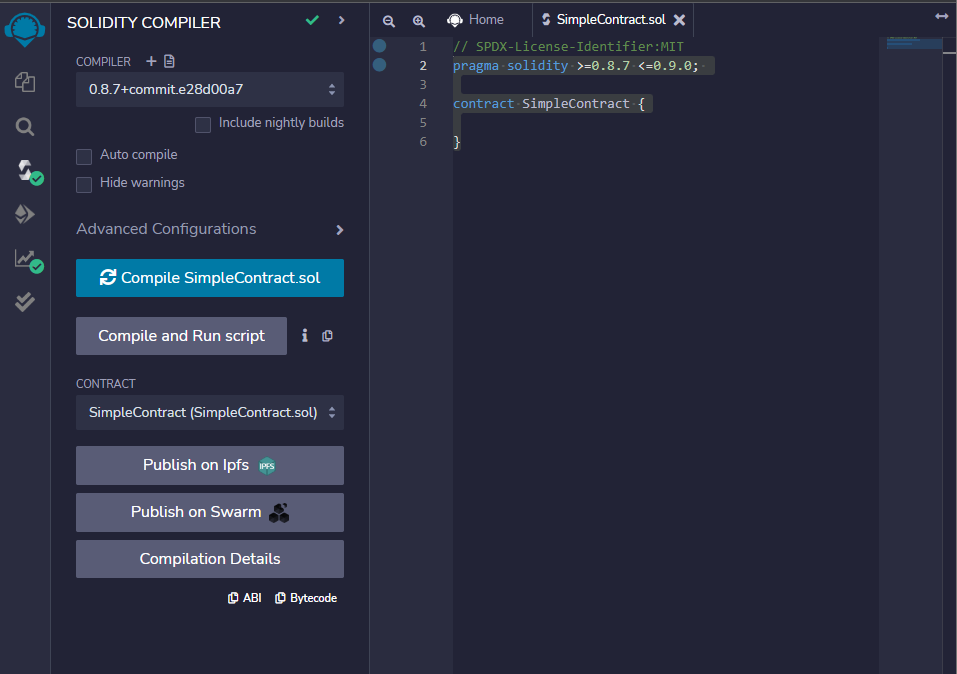
Declaring a variable and writing a function that stores a value
// SPDX-License-Identifier:MIT
pragma solidity >=0.8.7 <=0.9.0;
contract SimpleContract {
uint256 favoriteNumber;
function store(uint256 _favoriteNumber) public{
favoriteNumber = _favoriteNumber
}
UINT256 is a data type used in solidity for numbers that are 256 bytes. For further information about data types used in solidity. Please refer to the solidity documentation here, https://docs.soliditylang.org/en/latest/index.html
The Function Store is a very basic function that takes an argument and saves it to the variable declared above. The word Public indicates that the function is a public function and can be used anywhere in the class.
Now, when we hit save. The contract will be compiled, and that’s it you have successfully written your first smart contract in solidity that will function on the Ethereum blockchain.
Deploying our smart contract to a Virtual Machine to test it out
To deploy our contract, go to the deployment tab on the left,
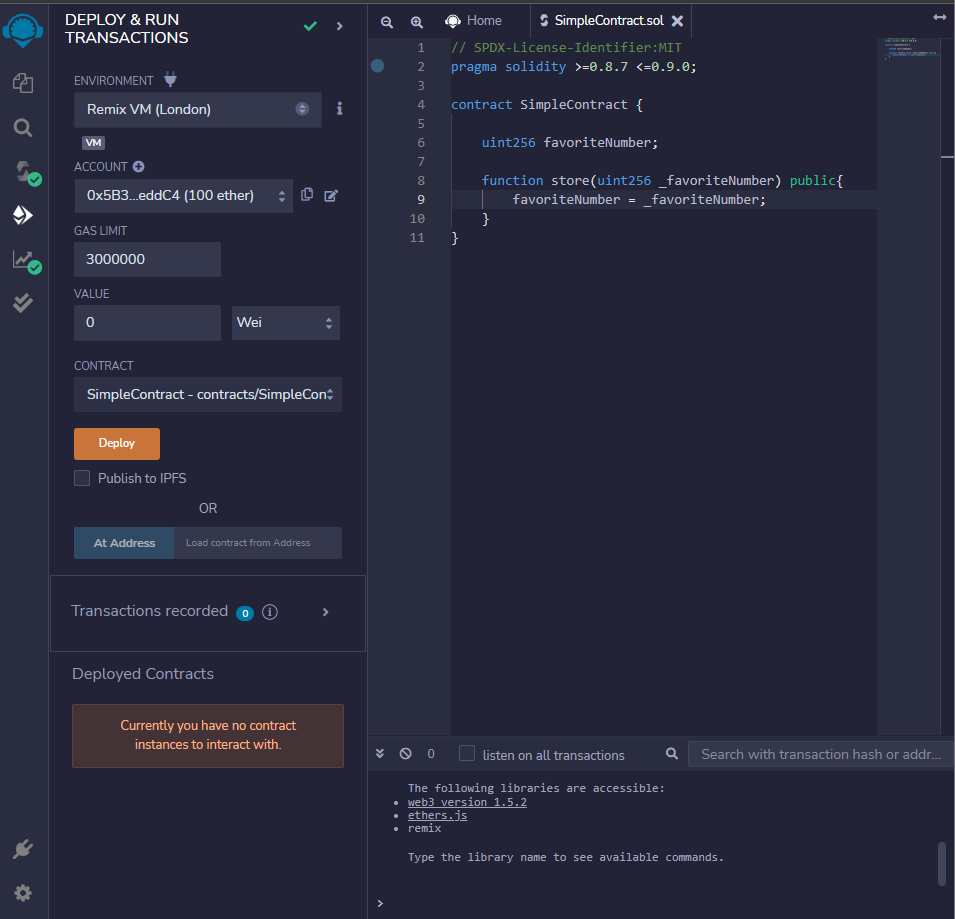
To Deploy our contract, we will be following these steps;
- Select a virtual machine form the environment
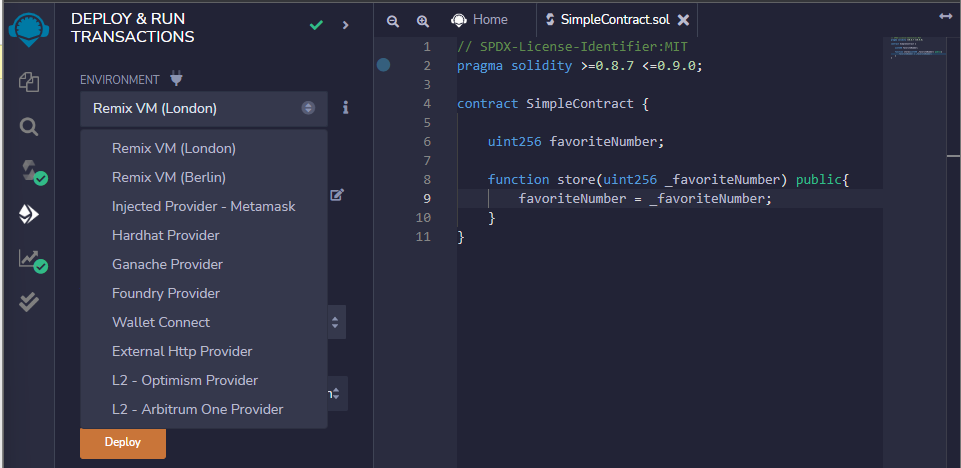
- Select an account from the fake accounts that are given in the account. When we run our smart contract on Virtual VM, we are given some fake accounts with 100 Ethereum in it. It is kind of similar to MetaMask to make real time Ethereum Transactions.
- Choose your smart contract that you have just written.
Hit the Button that Says Deploy
That’s it. You have just deployed your smart contract to a Virtual Machine and if someone knows the given address to your smart contract. They too can interact with it.
Interacting with our Smart contract
Your smart contract will appear in the same deployment tab on the left below once it is deployed.
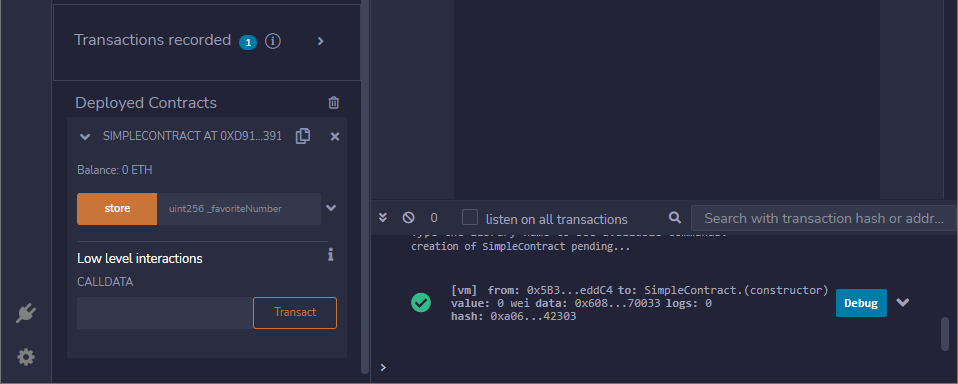
You will find your function Store that you wrote in your smart contract.
Go on and enter a value and hit Store.
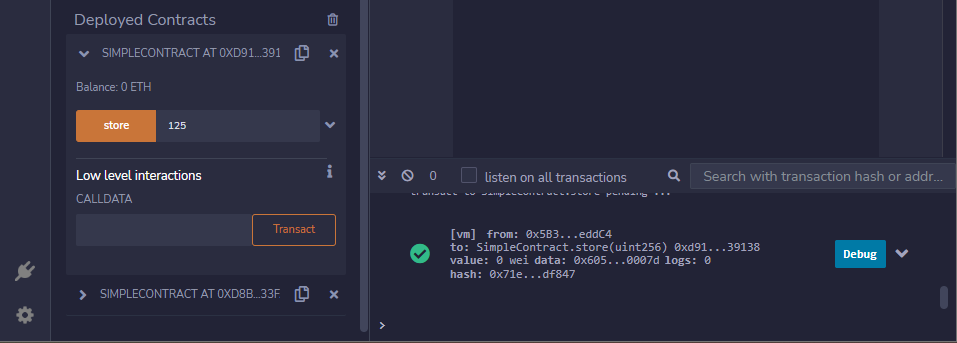
You will notice a smart contract appear at the very bottom of the page, and you will also notice a deduction from your fake account that you chose earlier to deploy the contract.
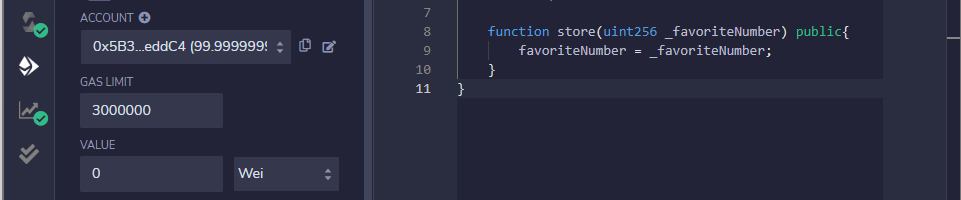
Which means, the value that you entered i.e., 125 is now stored on an Ethereum blockchain permanently and can never be deleted.
If you open the smart contract that appeared on the very bottom of the deployment tab, you will see a favoriteNumber button.
Now, store a new value again by entering a value in the text box, and click on the store button. To check the value, click on the favoriteNumber button, and it will show the number that you saved on Ethereum blockchain.
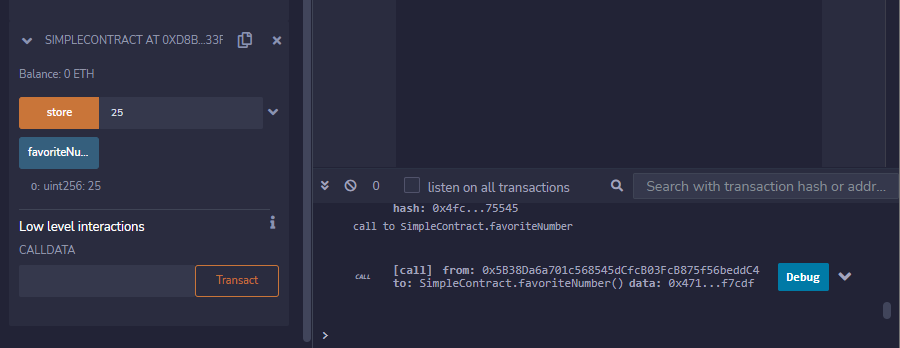
That is all that is needed to write a smart contract and deploy. Remember that simplest smart contract to deploy on a virtual machine using Remix, and fake Ethereum accounts. To further dive into solidity, always refer to the Solidity documentation to learn more about different use cases and usability of solidity in building different decentralized apps.
What is next?
Next step is to dive into solidity programming and its various use cases with smart contracts, DEFI, and NFTs. Solidity documentation is the best way to learn more about amazing features of solidity itself and how to implement more advanced and complicated smart contracts.